A Beginner’s Guide to Flutter: What You Need to Learn to Become a Professional Flutter Developer
Table of Content
Flutter revolutionized the way developers build cross-platform applications. With a single codebase, you can create apps for Android, iOS, the web, and desktop—all while maintaining a beautiful and consistent user interface.
But to harness the full power of Flutter, you need to know what to learn and how to progress from a beginner to a professional Flutter developer.
If you’re new to Flutter and want to master it, this guide will help you understand the key areas you need to focus on. Let’s break down everything a newcomer should learn to become proficient in Flutter.
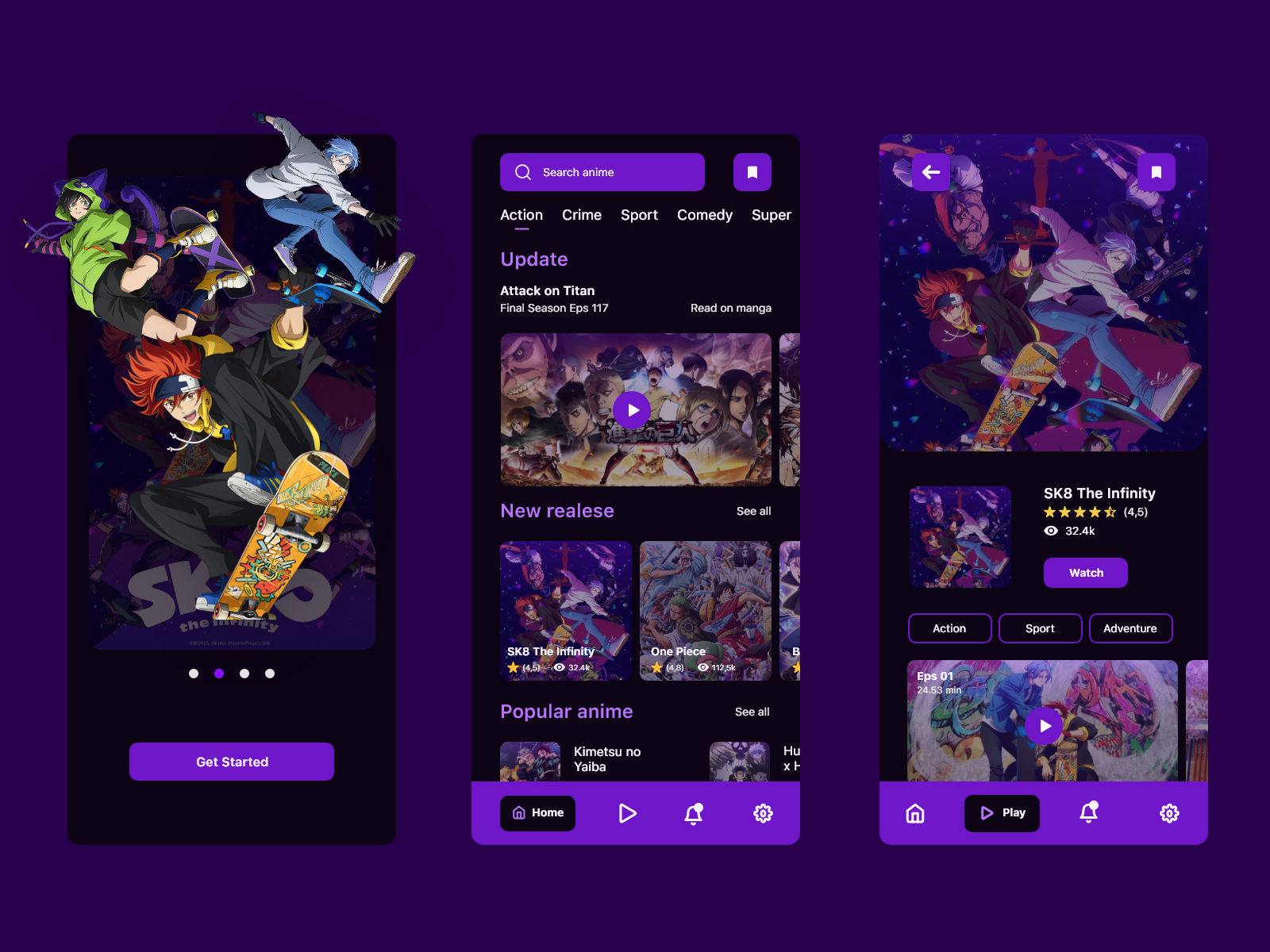
📌 1. Dart Programming Language
Flutter apps are built using Dart, a powerful and flexible language developed by Google. Learning Dart is the first step toward mastering Flutter.
Essential Dart Concepts:
- Variables and Data Types: Understand
String
,int
,double
,bool
, andList
types. - Functions: Learn to write reusable functions with and without return values.
- Object-Oriented Programming (OOP): Master classes, objects, inheritance, and interfaces.
- Null Safety: Understand Dart’s null safety features to write safer code.
- Asynchronous Programming: Learn
async
,await
,Future
, andStream
to handle asynchronous operations. - Collections: Work with lists, sets, and maps.
Example Code:
class User {
String name;
int age;
User(this.name, this.age);
void greet() {
print('Hello, $name!');
}
}
void main() {
var user = User('Alice', 25);
user.greet();
}
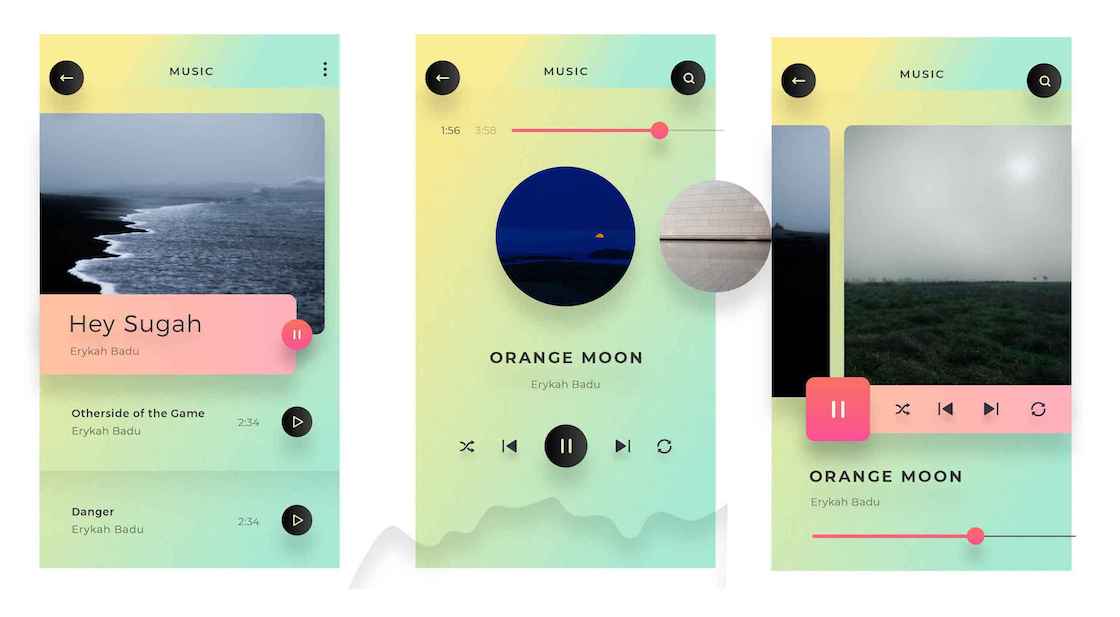
🧱 2. Flutter Fundamentals
Core Flutter Concepts to Master:
- Widgets:
- StatelessWidget: For static UIs that don’t change over time.
- StatefulWidget: For dynamic UIs that update based on user interaction or data changes.
- InheritedWidget: For managing state across a widget tree.
- Widget Tree:
- Understand the hierarchical structure of widgets.
- Learn about the build context and how widgets are rendered.
- Layouts:
- Master layout widgets like
Row
,Column
,Stack
, andContainer
. - Use
Flex
andExpanded
to create flexible UIs.
- Master layout widgets like
- Navigation:
- Implement basic and advanced navigation using
Navigator
and routing.
- Implement basic and advanced navigation using
- Themes:
- Customize your app’s look and feel using
ThemeData
.
- Customize your app’s look and feel using
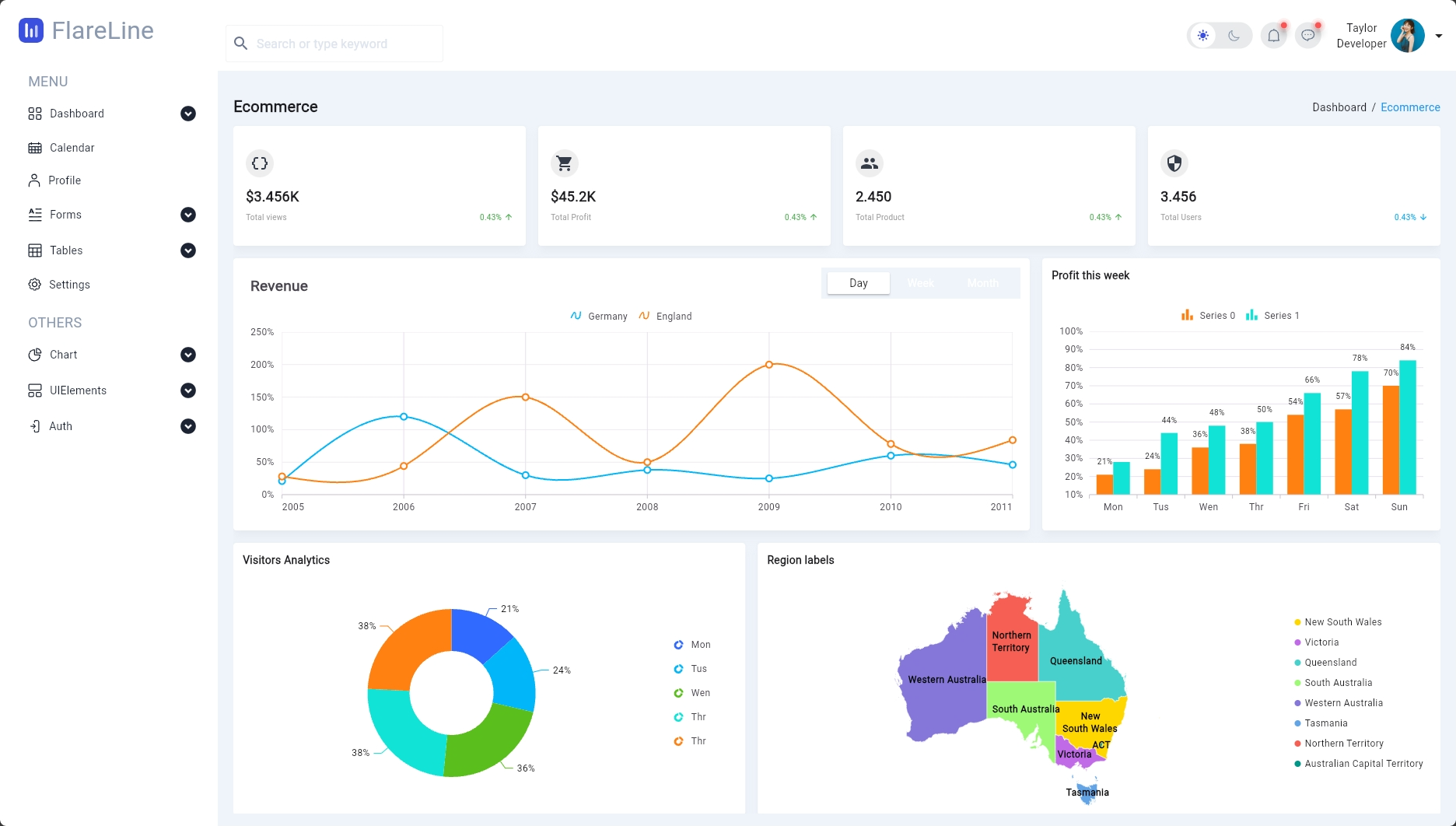
🎨 3. Creating Beautiful UIs
Flutter is all about creating expressive UIs. To be a professional developer, you need to learn how to build attractive and responsive user interfaces.
UI Concepts to Learn:
- Material Design: Flutter offers Material Design widgets like
AppBar
,Card
, andFloatingActionButton
. - Cupertino Design: For iOS-style widgets (
CupertinoButton
,CupertinoNavigationBar
). - Responsive Design: Use
LayoutBuilder
andMediaQuery
to adapt your app to different screen sizes. - Animations: Add motion with Flutter’s
Tween
,AnimationController
, andHero
widgets.
Example Responsive Layout:
LayoutBuilder(
builder: (context, constraints) {
if (constraints.maxWidth > 600) {
return Text('Large Screen');
} else {
return Text('Small Screen');
}
},
);
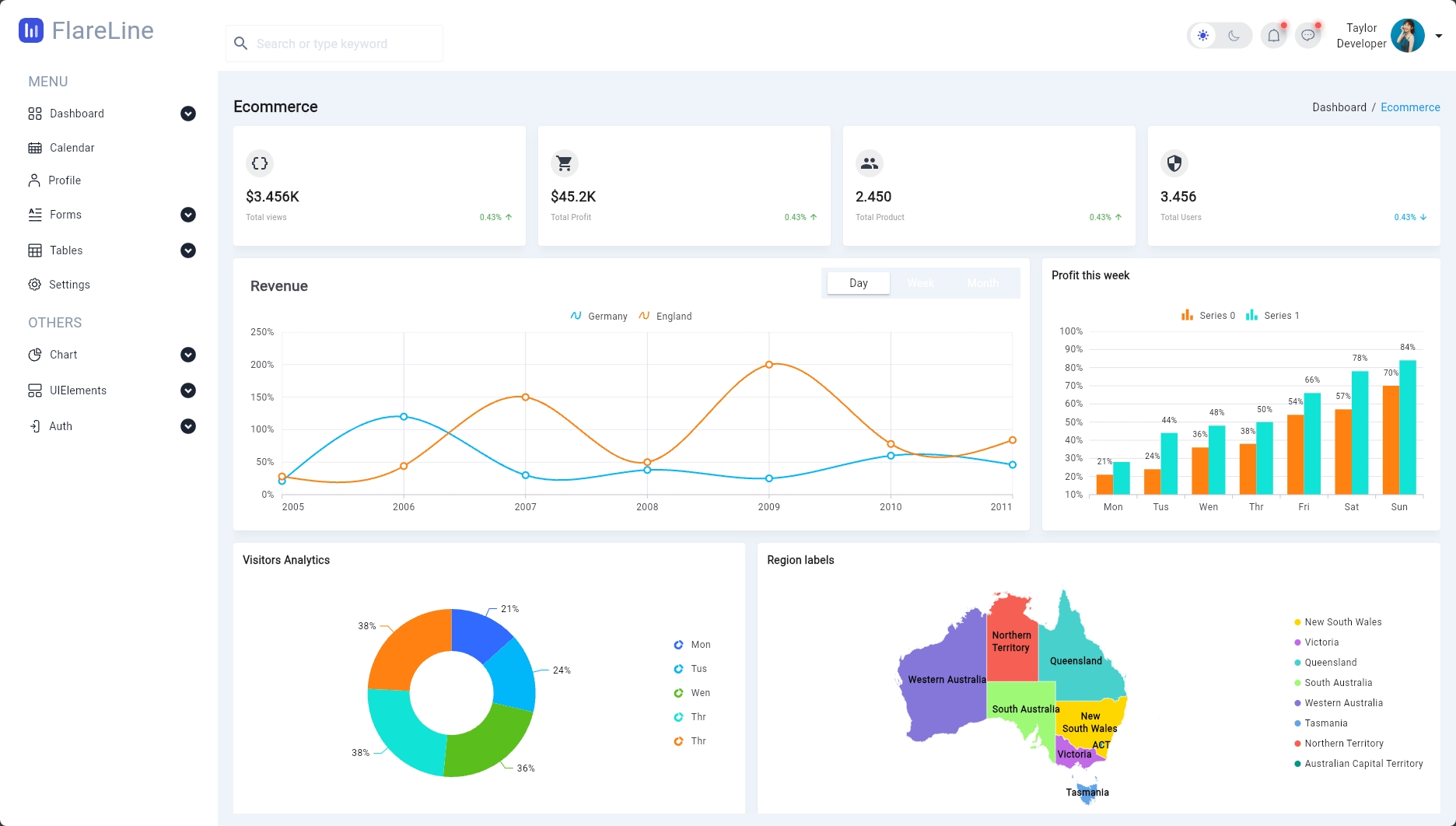
🔄 4. State Management
Managing the state of your app is one of the most important skills for a Flutter developer.
There are multiple approaches to state management, each suitable for different use cases.
Popular State Management Solutions:
- Provider:
Simple and beginner-friendly. Ideal for small to medium-sized apps. - Riverpod:
Modern, robust, and a recommended alternative to Provider. - Bloc (Business Logic Component):
For complex apps requiring a clear separation of business logic and UI. - GetX:
Minimalistic and offers state management, routing, and dependency injection. - Redux:
Inspired by React, suitable for apps requiring a unidirectional data flow.
Example with Provider:
class CounterModel extends ChangeNotifier {
int _count = 0;
int get count => _count;
void increment() {
_count++;
notifyListeners();
}
}
📡 5. Working with APIs and Databases
Key Skills to Learn:
- REST APIs:
- Use the
http
package to make API calls. - Handle JSON responses and errors.
- Use the
- Local Storage:
- Use
sqflite
for SQLite databases. - Use
Hive
for NoSQL storage.
- Use
- Firebase Integration:
- Authentication, Firestore database, and real-time updates.
Example API Call:
import 'package:http/http.dart' as http;
Future<void> fetchData() async {
final response = await http.get(Uri.parse('https://jsonplaceholder.typicode.com/posts'));
if (response.statusCode == 200) {
print(response.body);
} else {
throw Exception('Failed to load data');
}
}
📲 6. Cross-Platform Deployment
Flutter allows you to deploy your apps on multiple platforms:
- Mobile: Android and iOS
- Web: Single-page applications (SPA) and progressive web apps (PWA)
- Desktop: Windows, macOS, and Linux apps
Understanding how to adapt your Flutter code for each platform will make you a versatile developer.
🛠 7. Debugging and Performance Optimization
Essential Tools and Techniques:
- Flutter DevTools:
- Inspect widget trees, monitor performance, and debug your apps.
- Logging and Error Handling:
- Use
print
statements,debugPrint
, and error-handling mechanisms.
- Use
- Performance Optimization:
- Minimize widget rebuilds.
- Optimize images and assets.
- Analyze performance using Flutter’s built-in profiling tools.
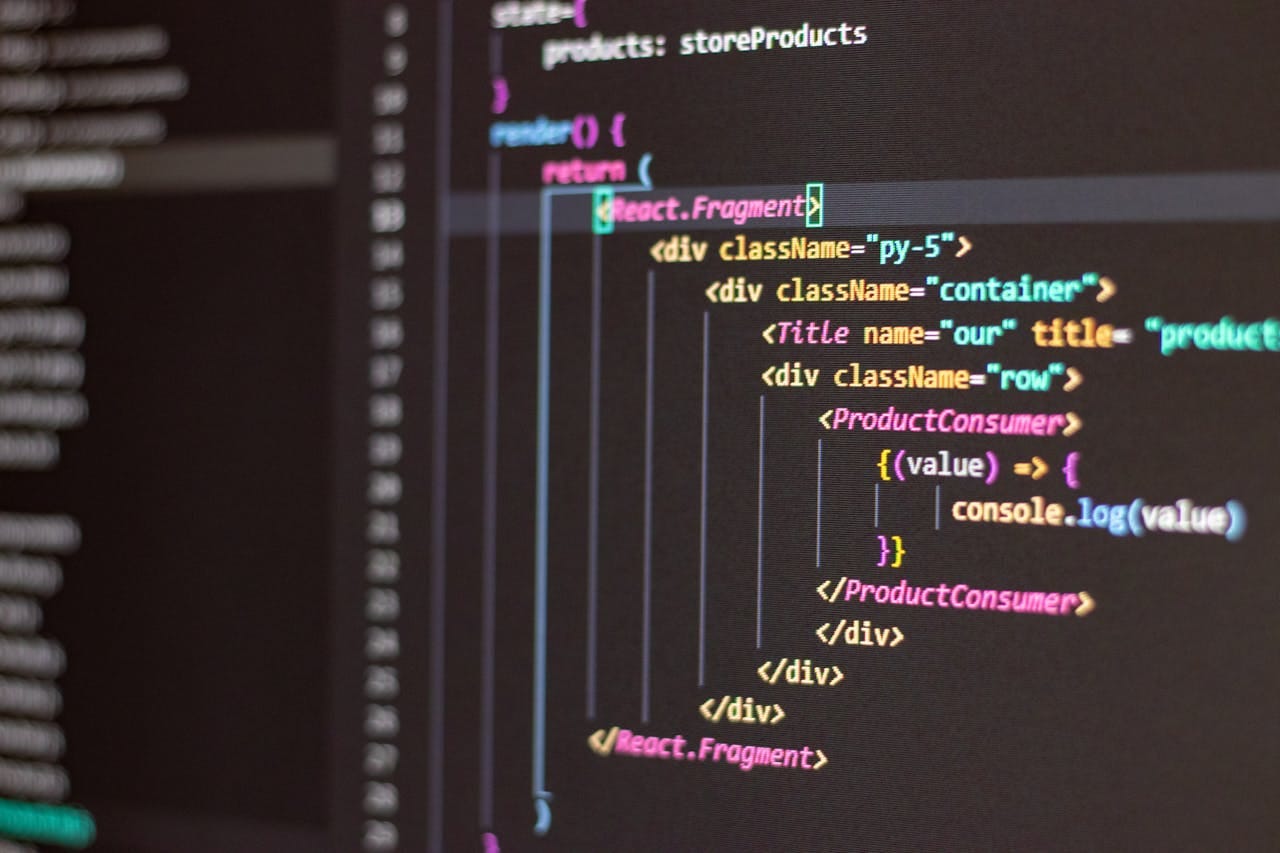
🚀 8. Advanced Flutter Concepts
Once you’re comfortable with the basics, explore advanced topics:
- Platform Channels:
Integrate native code (Swift, Kotlin) with Flutter. - Custom Painters:
Create custom graphics and animations. - Isolates:
Perform heavy computations in the background. - CI/CD Pipelines:
Automate builds and deployments with tools like GitHub Actions and Codemagic.
🌐 9. Community and Continuous Learning
Where to Stay Updated:
- Flutter Community: Join forums, Discord channels, and Reddit communities.
- Open Source: Contribute to Flutter packages on GitHub.
- Events: Attend Flutter meetups and conferences like Flutter Engage.
🎯 Conclusion
Becoming a professional Flutter developer is a journey of continuous learning. By mastering Dart, Flutter fundamentals, state management, and advanced techniques, you’ll be well on your way to creating incredible apps.
Stay curious, build projects, and engage with the community—and before long, you'll be a Flutter pro! 🚀