The AI-Database Connection: Transforming Raw Data into Intelligent Solutions. Complete Tutorial with Next.js, MySQL and OpenAI
Why and How to Connect AI to Your Databases: A Comprehensive Guide
From digital marketplaces to medical institutions and information analysis, these intelligent systems offer invaluable insights and streamline operations, revolutionizing decision-making processes and operational efficiency. However, to truly harness the potential of these cognitive technologies, a symbiotic relationship with your information repositories is crucial.
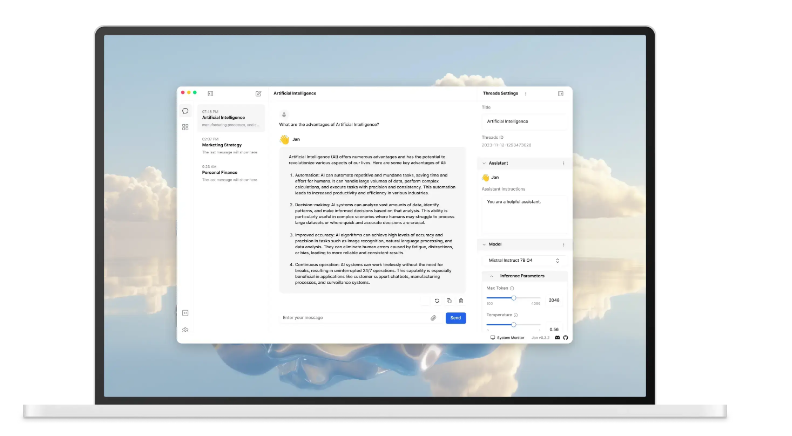
This comprehensive guide will navigate you through the rationale and methodology of establishing a connection between advanced cognitive systems, such as conversational interfaces, and your data storage solutions. We'll explore how to leverage modern web development frameworks like React and Next.js to craft an intuitive interface for seamless interaction with these intelligent systems.
The benefits of this integration are far-reaching. Smarter decision-making becomes possible as AI algorithms process and interpret data at speeds and scales beyond human capability, offering insights that can inform everything from product development to marketing strategies. Real-time insights allow businesses to respond quickly to changing market conditions, customer preferences, or operational issues, enabling a level of agility that can be a significant competitive advantage. Moreover, the ability to deliver more personalized user experiences can dramatically improve customer satisfaction and loyalty, as AI can tailor interactions based on individual preferences, behavior patterns, and historical data.

By leveraging modern web development technologies like React and Next.js, along with powerful AI platforms such as OpenAI, businesses can create seamless AI-driven interfaces that work harmoniously with their existing data infrastructure.
By the end of this journey, you'll have a thorough understanding of how to bridge the gap between your data and cutting-edge cognitive technologies, opening up a world of possibilities for innovation and growth in your field. Whether you're a seasoned technologist or a curious newcomer, this article will provide you with the knowledge and tools to embark on this transformative integration.
Why Connect AI to Your Database?
1- Enhanced Decision-Making:
By connecting AI to your database, you can harness the power of advanced question-answering, trend analysis, and predictive analytics. This integration enables more informed decision-making based on historical data, rather than relying solely on intuition.
AI can process vast amounts of information quickly, identifying patterns and insights that might be missed by human analysis alone. This data-driven approach enhances strategic planning and operational efficiency across various business functions.
2- Improved Efficiency:
Automating AI-database interactions streamlines processes, enabling real-time responses to queries. This automation fetches data like inventory levels, sales reports, and user behaviors instantly, significantly accelerating workflows and reducing manual effort across various business operations.
4- Personalized User Experience:
AI can tailor responses based on the data it has access to, creating a highly personalized experience. For example, in an e-commerce setting, it can generate product recommendations by analyzing a user's purchase history and browsing behavior.
Similarly, in a healthcare application, AI can dynamically respond to patient inquiries by fetching relevant medical records and treatment plans from the database, ensuring accurate and personalized health advice.
How to Connect AI to Your Database
To connect AI, such as OpenAI's GPT models, to a database, we'll break down the process into clear, manageable steps. This integration allows for powerful data-driven interactions and insights.
We'll use React for building a dynamic user interface and Next.js as our full-stack framework, which will handle both frontend and backend operations. This combination provides a robust foundation for creating an interactive AI-powered application that can communicate with your database.
The frontend, built with React, will serve as the user-facing interface where queries can be input and responses displayed. Next.js will facilitate server-side rendering and API route creation, making it easier to handle database operations and AI interactions on the backend. We'll use these technologies to create an API that interacts with both the AI model and your database, forming a bridge between user inputs, stored data, and AI-generated responses.
Throughout this tutorial, we'll cover setting up your development environment, creating necessary API endpoints, implementing database connections, and integrating the AI model.
We'll also discuss best practices for secure and efficient data handling. Here's a detailed walkthrough of how to set up this powerful integration:
Step 1: Choose Your AI Model
For this example, we'll use OpenAI's GPT API. OpenAI offers a flexible API that integrates seamlessly into your application. We'll build a custom UI with React to interact with it.
Step 2: Setup Your Database
For this tutorial, we’ll use MySQL as our database, although other SQL or NoSQL databases could be used as well.
Install MySQL locally or use a cloud-hosted solution like AWS RDS or Azure SQL.
sudo apt-get update
sudo apt-get install mysql-server
Create a Sample Database: Create a database and a table for this example:
CREATE DATABASE ai_database;
USE ai_database;
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255),
email VARCHAR(255),
age INT
);
Populate with Sample Data: Populate the database with some mock data.
INSERT INTO users (name, email, age) VALUES ('John Doe', 'john@example.com', 29);
Step 3: Set Up the Backend with Next.js
Next.js offers full-stack capabilities, allowing us to create both frontend and backend logic.
Create an API Route to Fetch DataIn Next.js, create a new file under pages/api/
to handle the backend logic of fetching data from the database.
// pages/api/fetchData.js
import mysql from 'mysql2/promise';
export default async function handler(req, res) {
const connection = await mysql.createConnection({
host: 'localhost',
user: 'root',
database: 'ai_database'
});
const [rows] = await connection.execute('SELECT * FROM users');
res.status(200).json({ data: rows });
}
Install Dependencies: Install the necessary dependencies to connect to MySQL:
npm install mysql2 openai
Create a New Next.js Project: In your terminal, run:
npx create-next-app@latest ai-database-connector
cd ai-database-connector
Step 4: Integrate AI (OpenAI) in Your React Component
Create API Route to Interact with GPT: Now, create an API route to interact with OpenAI’s API and provide an answer based on user input.
// pages/api/aiQuery.js
import { Configuration, OpenAIApi } from 'openai';
const configuration = new Configuration({
apiKey: process.env.OPENAI_API_KEY,
});
const openai = new OpenAIApi(configuration);
export default async function handler(req, res) {
const { message, data } = req.body;
const prompt = `Based on the data: ${JSON.stringify(data)}, answer this question: ${message}`;
const response = await openai.createCompletion({
model: 'gpt-3.5-turbo',
prompt,
max_tokens: 150,
});
res.status(200).json({ reply: response.data.choices[0].text });
}
Create the AI Chat Component: In this step, we’ll create a simple React component where users can input a query, and the response will be provided by ChatGPT based on data from the database.
// components/ChatComponent.js
import { useState } from 'react';
export default function ChatComponent() {
const [message, setMessage] = useState('');
const [response, setResponse] = useState('');
const handleQuery = async () => {
const res = await fetch('/api/fetchData');
const data = await res.json();
const aiResponse = await fetch('/api/aiQuery', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ message, data })
});
const aiData = await aiResponse.json();
setResponse(aiData.reply);
};
return (
<div>
<textarea
value={message}
onChange={(e) => setMessage(e.target.value)}
placeholder="Ask a question..."
/>
<button onClick={handleQuery}>Send</button>
<p>{response}</p>
</div>
);
}
Step 5: Deploy and Test
- Test the Integration: Now head to your browser and type:
http://localhost:3000
and try querying the database via the ChatGPT-powered interface. You should see responses based on the data in your MySQL database.
Run the Project: Start your Next.js development server:
npm run dev
Set Environment Variables: Add your OpenAI API key in a .env.local
file:
OPENAI_API_KEY=your_openai_api_key
Conclusion
Connecting AI to your database opens up a world of possibilities for creating interactive and intelligent applications. This integration has the potential to revolutionize how businesses operate, regardless of their size or industry.
For large-scale enterprises, AI-database integration can streamline complex processes, analyze vast amounts of data in real-time, and provide actionable insights that drive strategic decision-making.
Small businesses, on the other hand, can leverage this technology to compete more effectively by personalizing customer experiences, optimizing inventory management, and identifying market trends that might otherwise go unnoticed.
Further Reading and References
- Connecting ChatGPT to Your SQL Database
- How to Use Today’s AI With Your Own Data
- MIT Researchers Introduce Generative AI Databases
- How to Query Your Database With AI: A Comprehensive Guide
With these resources, you can dive deeper into optimizing the connection between AI and your data to unlock new business potentials.