Pandas is an incredibly popular open-source data manipulation and analysis library for Python. It has gained immense popularity due to its ability to simplify complex data handling tasks.
With Pandas, you can effortlessly work with various data structures and leverage a wide range of data analysis tools to manipulate and explore structured data. Whether you need to clean messy data, transform datasets, explore patterns, or create visually appealing visualizations, Pandas has got you covered.
One of the key strengths of Pandas is its versatility. It empowers users to perform a multitude of operations on datasets, giving them the freedom to filter, sort, aggregate, merge, and reshape data as desired. This flexibility makes it an invaluable tool in various domains, including data science, machine learning, and data analysis workflows.
In the realm of data science, Pandas serves as a fundamental building block for conducting rigorous analyses and extracting meaningful insights from raw data. Its user-friendly syntax and extensive functionality enable data scientists to efficiently explore and manipulate datasets, allowing for seamless integration with other libraries such as NumPy, Matplotlib, and Scikit-learn.
Furthermore, Pandas plays a vital role in machine learning workflows by facilitating data preprocessing, feature engineering, and model evaluation. It simplifies the process of preparing data for training and testing machine learning models, ensuring that the data is in the right format and ready for analysis.
The ability to handle large datasets efficiently makes Pandas an indispensable tool for machine learning practitioners.
In summary, Pandas is a game-changing library that revolutionizes the way data is handled and analyzed in Python. Its rich feature set, intuitive interface, and extensive community support have made it the go-to choice for data manipulation and analysis tasks. Whether you are a beginner or an experienced data professional, Pandas is an essential tool in your toolkit.
Here are some code snippets for the "DataFrame Tricks with Pandas" tutorial:
1- Sorting a DataFrame by a specific column
df.sort_values('column_name', inplace=True)
2- Creating a new column based on conditions
df['new_column'] = np.where(df['condition'], df['value_if_true'], df['value_if_false'])
3- Filtering rows based on multiple conditions
filtered_df = df[(df['condition1']) & (df['condition2'])]
4- Grouping and aggregating data
grouped_df = df.groupby('column_name').agg({'column1': 'sum', 'column2': 'mean'})
5- Handling missing values
df.dropna() # drop rows with missing values
df.fillna(value) # fill missing values with a specific value
6- Merging multiple DataFrames
merged_df = pd.merge(df1, df2, on='common_column')
7- Reshaping data using pivot tables
pivot_table = df.pivot_table(index='index_column', columns='columns_column', values='values_column', aggfunc='mean')
8- Applying a function to a column
df['column'] = df['column'].apply(lambda x: function(x))
9- Working with datetime data
df['date_column'] = pd.to_datetime(df['date_column'])
df['year'] = df['date_column'].dt.year
10- Visualizing data using Matplotlib
import matplotlib.pyplot as plt
df.plot(kind='bar', x='x_column', y='y_column')
plt.show()
More
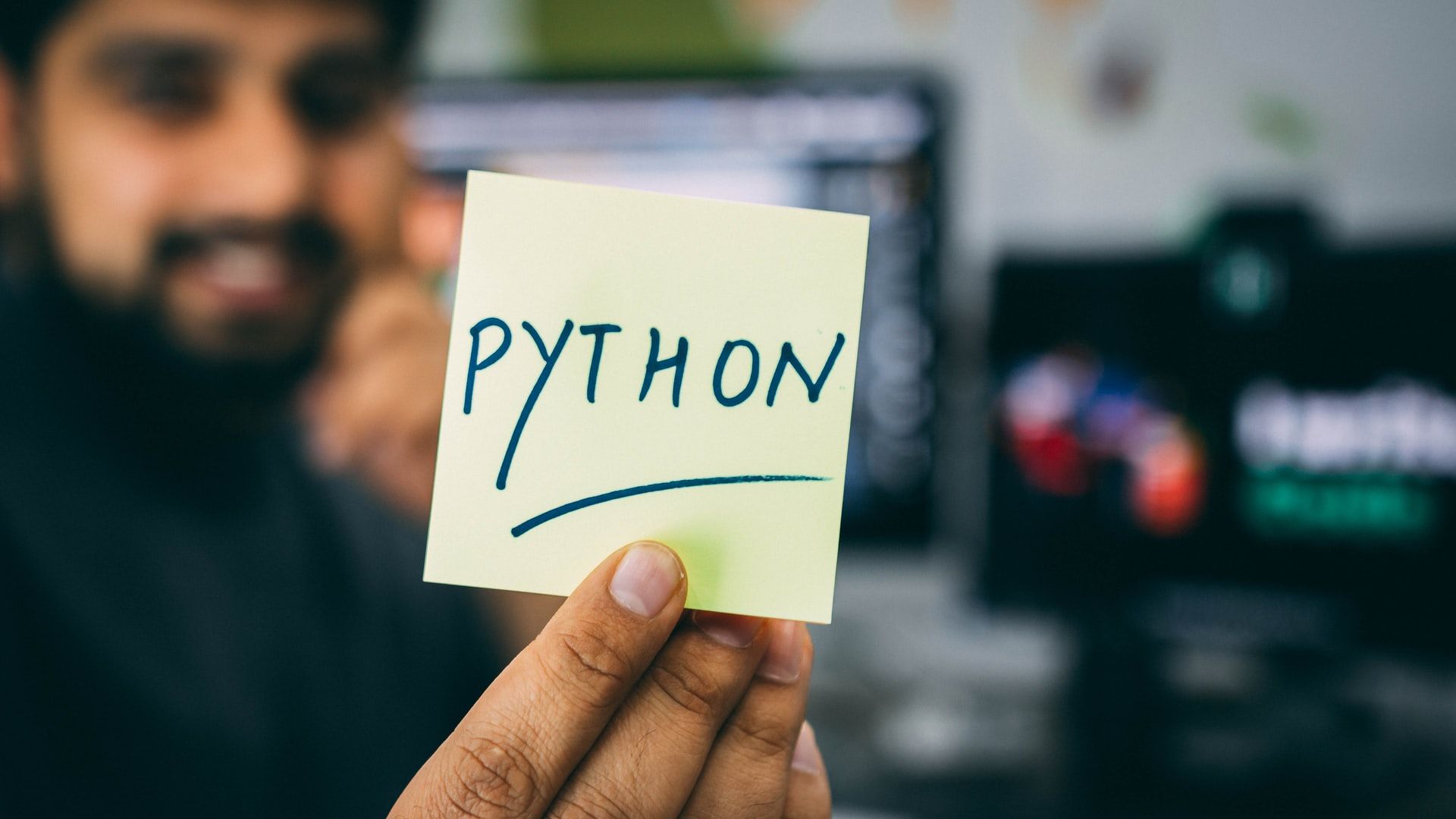