Create Reactive Todo List with Svelte and Pocketbase
Table of Content
Pocketbase is an open-source backend designed for simplicity, offering real-time databases, file storage, authentication, and more. It’s lightweight and can be easily integrated with frontend frameworks like Svelte.
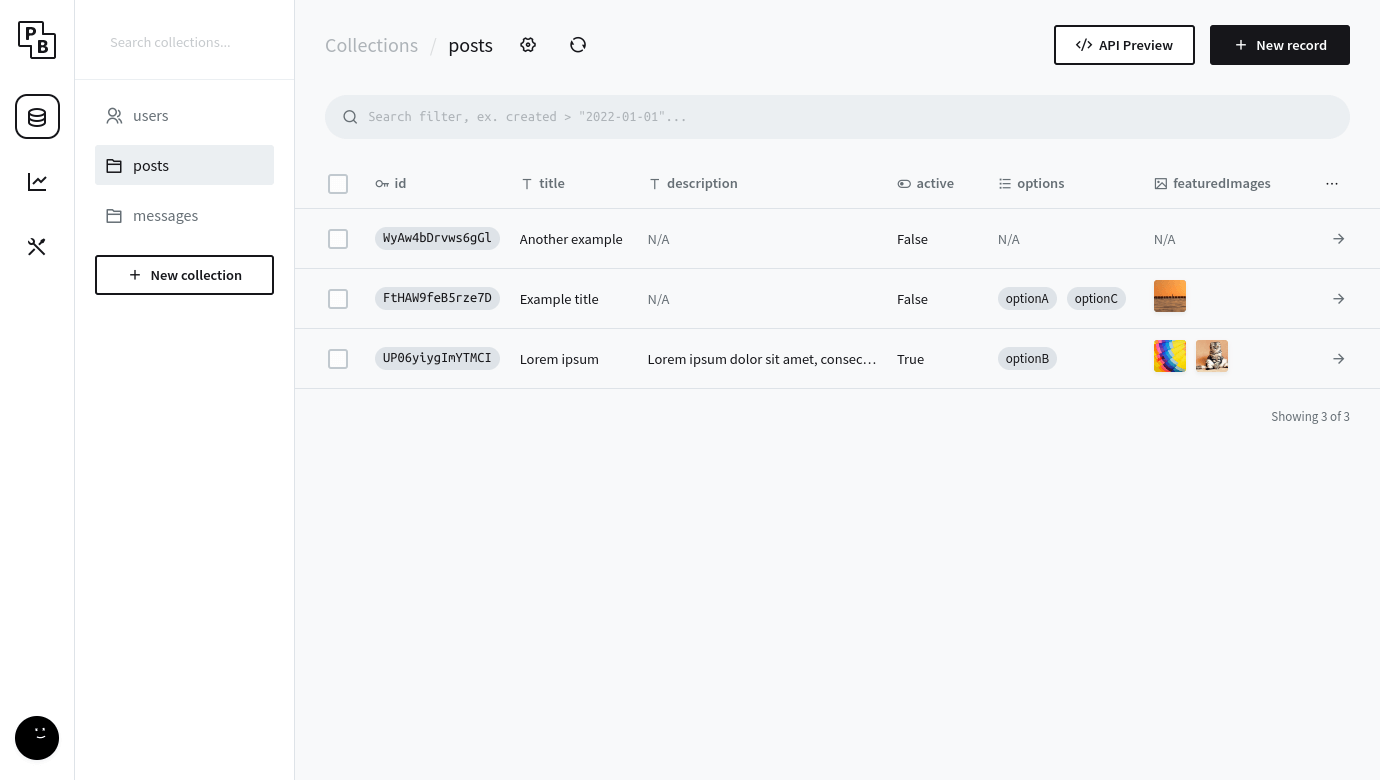
Download Pocketbase
Download the Pocketbase binary from the official website. Extract it and navigate to the directory where you extracted the file.
Set Up Your Database
Open the Pocketbase admin UI by navigating to http://localhost:8090/_
in your browser. Create a new collection called todos
with the following fields:Create another collection called users
with the default fields (email
, password
).
title
: Text field (required)completed
: Boolean field (default tofalse
)
Start the Pocketbase Server
Run the Pocketbase server with the following command:
./pocketbase serve
Pocketbase will start and be accessible at http://localhost:8090/_
.
Install with Docker
Alternatively you can install Pocketbase using Docker and Docker Compose.
Setting Up Svelte with Pocketbase
Install Pocketbase SDK
Install the Pocketbase JavaScript SDK to interact with your Pocketbase backend:
npm install pocketbase
Initialize a Svelte Project
If you don’t already have a Svelte project, create one:
npx degit sveltejs/template svelte-pocketbase-todo
cd svelte-pocketbase-todo
npm install
npm run dev
Implementing Login Functionality
Integrate Login with the Main Application
In your App.svelte
, conditionally render the Login
component based on the user's authentication status:
<script>
import Login from './Login.svelte';
import TodoList from './TodoList.svelte';
import PocketBase from 'pocketbase';
import { onMount } from 'svelte';
let pb;
let isAuthenticated = false;
onMount(() => {
pb = new PocketBase('http://localhost:8090');
isAuthenticated = pb.authStore.isValid;
});
pb.authStore.onChange(() => {
isAuthenticated = pb.authStore.isValid;
});
</script>
{#if isAuthenticated}
<TodoList />
{:else}
<Login />
{/if}
Create a Login Component
Create a new Svelte component Login.svelte
:
<script>
import PocketBase from 'pocketbase';
import { onMount } from 'svelte';
let email = '';
let password = '';
let pb;
onMount(() => {
pb = new PocketBase('http://localhost:8090');
});
async function login() {
try {
const authData = await pb.collection('users').authWithPassword(email, password);
console.log('Logged in successfully:', authData);
} catch (err) {
console.error('Failed to login:', err);
}
}
</script>
<div>
<h2>Login</h2>
<input type="email" placeholder="Email" bind:value={email} />
<input type="password" placeholder="Password" bind:value={password} />
<button on:click={login}>Login</button>
</div>
Creating the Todo List with CRUD Operations
Displaying and Managing Todos
In the TodoList.svelte
component, you’ll be able to display all the todos, add new todos, update their completion status, and delete them.
Create the TodoList Component
Create a new Svelte component TodoList.svelte
:
<script>
import { onMount } from 'svelte';
import PocketBase from 'pocketbase';
let todos = [];
let newTodoTitle = '';
let pb;
onMount(async () => {
pb = new PocketBase('http://localhost:8090');
await fetchTodos();
});
async function fetchTodos() {
const records = await pb.collection('todos').getFullList();
todos = records;
}
async function addTodo() {
const newTodo = await pb.collection('todos').create({ title: newTodoTitle, completed: false });
todos = [...todos, newTodo];
newTodoTitle = '';
}
async function toggleTodoCompletion(todo) {
todo.completed = !todo.completed;
await pb.collection('todos').update(todo.id, { completed: todo.completed });
}
async function deleteTodo(id) {
await pb.collection('todos').delete(id);
todos = todos.filter(todo => todo.id !== id);
}
</script>
<h1>Todo List</h1>
<input placeholder="New Todo" bind:value={newTodoTitle} />
<button on:click={addTodo}>Add Todo</button>
<ul>
{#each todos as todo}
<li>
<input type="checkbox" bind:checked={todo.completed} on:change={() => toggleTodoCompletion(todo)} />
{todo.title}
<button on:click={() => deleteTodo(todo.id)}>Delete</button>
</li>
{/each}
</ul>
Conclusion
With this tutorial, you’ve learned how to integrate Pocketbase with Svelte to build a simple CRUD todo list application with authentication.
Pocketbase provides a flexible and powerful backend solution that pairs well with Svelte’s reactive and component-based architecture.
This setup can be expanded with additional features such as user registration, data validation, and real-time updates.