Data Visualization in Julia Language for Data Engineers
Data visualization is an essential aspect of data analysis, enabling you to understand and communicate your data's underlying patterns and insights. Julia offers several powerful packages for creating various types of visualizations, from basic plots to complex interactive charts.
In this tutorial will introduce you to some of the most popular Julia visualization packages and provide tips for effective data visualization.
Popular Data Visualization Packages in Julia
1. Plots.jl
Summary: A versatile plotting package in Julia that supports multiple backends, enabling users to create a wide range of visualizations with ease. It provides a simple syntax and powerful customization options.
GitHub: Plots.jl
2. Gadfly.jl
Summary: Gadfly.jl is a plotting and data visualization system in Julia based on the grammar of graphics, allowing users to create complex and aesthetic plots with a declarative syntax.
GitHub: Gadfly.jl
3. Makie.jl
Summary: A high-performance and flexible plotting package in Julia, Makie.jl supports interactive 2D and 3D visualizations, making it ideal for scientific computing and complex data visualizations.
GitHub: Makie.jl
4. VegaLite.jl
Summary: VegaLite.jl provides an interface to Vega-Lite, a high-level grammar of interactive graphics, enabling users to create sophisticated, interactive visualizations with concise and expressive syntax.
GitHub: VegaLite.jl
5. PyPlot.jl
Summary: A Julia interface to the popular Python matplotlib library, PyPlot.jl allows for the creation of a wide variety of static, animated, and interactive plots with extensive customization options.
GitHub: PyPlot.jl
6. GR.jl
Summary: GR.jl is a powerful, high-performance visualization package in Julia, providing a wide range of plotting capabilities with minimal dependencies, making it suitable for both simple and complex plots.
GitHub: GR.jl
These summaries and links should provide a concise overview of each plotting library along with direct access to their GitHub repositories for more detailed information.
Installing Packages
To get started, install the necessary packages using Julia's package manager.
using Pkg
Pkg.add(["Plots", "Gadfly", "Makie", "VegaLite"])
Basic Plotting with Plots.jl
Plots.jl
is a powerful and flexible plotting package that supports multiple backends like GR, PyPlot, Plotly, and more. Here's a basic introduction to using Plots.jl
.
using Plots
# Create a simple line plot
x = 1:10
y = rand(10)
plot(x, y, title="Line Plot", xlabel="X-Axis", ylabel="Y-Axis", label="Random Data")
# Save the plot
savefig("line_plot.png")
Customizing Plots
You can customize various aspects of your plots in Plots.jl
, such as colors, markers, and legends.
# Create a scatter plot with customized markers and colors
scatter(x, y, title="Scatter Plot", xlabel="X-Axis", ylabel="Y-Axis", label="Random Data",
marker=:circle, color=:blue, legend=:topright)
Advanced Plotting with Gadfly.jl
Gadfly.jl
is inspired by the Grammar of Graphics and provides a declarative syntax for creating complex plots.
using Gadfly
# Create a basic plot
plot(x=1:10, y=rand(10), Geom.point, Geom.line,
Theme(title="Gadfly Plot", xlabel="X-Axis", ylabel="Y-Axis"))
Interactive Plots with Makie.jl
Makie.jl
is designed for high-performance and interactive plotting.
using GLMakie
# Create an interactive plot
x = 1:10
y = rand(10)
fig = Figure(resolution = (800, 600))
ax = Axis(fig[1, 1], title="Makie Plot", xlabel="X-Axis", ylabel="Y-Axis")
lines!(ax, x, y, linewidth=2)
scatter!(ax, x, y, markersize=10, color=:red)
fig
Data-Driven Visualizations with VegaLite.jl
VegaLite.jl
provides an interface to the Vega-Lite visualization grammar, allowing for concise and declarative plotting.
using VegaLite
# Create a simple bar chart
data = (category=["A", "B", "C", "D"], value=[5, 3, 6, 7])
@vlplot(:bar, data, x=:category, y=:value, color=:category)
Example: Visualizing a Dataset
Let's visualize a dataset using different Julia packages.
Loading the Data
First, load a dataset using the CSV
and DataFrames
packages.
using CSV
using DataFrames
df = CSV.File("data.csv") |> DataFrame
Plotting with Plots.jl
using Plots
# Line plot of two columns
plot(df[:column1], df[:column2], title="Line Plot", xlabel="Column 1", ylabel="Column 2", label="Data Line")
Plotting with Gadfly.jl
using Gadfly
# Scatter plot of two columns
plot(df, x=:column1, y=:column2, Geom.point,
Theme(title="Gadfly Scatter Plot", xlabel="Column 1", ylabel="Column 2"))
Plotting with Makie.jl
using GLMakie
# Line and scatter plot
fig = Figure(resolution = (800, 600))
ax = Axis(fig[1, 1], title="Makie Plot", xlabel="Column 1", ylabel="Column 2")
lines!(ax, df[:column1], df[:column2], linewidth=2)
scatter!(ax, df[:column1], df[:column2], markersize=10, color=:blue)
fig
Plotting with VegaLite.jl
using VegaLite
# Bar chart of two columns
@vlplot(:bar, df, x=:column1, y=:column2, color=:column1)
Tips for Effective Data Visualization
- Choose the Right Type of Chart: Select a chart type that best represents the data and the message you want to convey.
- Simplify: Avoid clutter by keeping your visualizations simple and focused.
- Use Colors Wisely: Use colors to highlight important data points but avoid using too many colors that can be distracting.
- Label Clearly: Ensure your axes, legends, and titles are clearly labeled to make the plot easily understandable.
- Provide Context: Add necessary context, such as annotations or reference lines, to help interpret the data.
- Interactivity: Where possible, use interactive plots to allow users to explore the data further.
Conclusion
Julia offers a variety of powerful tools for data visualization, catering to different needs and preferences. Whether you need simple static plots or complex interactive visualizations, Julia's ecosystem has you covered.
By following best practices and using the right tools, you can create effective and insightful visualizations to communicate your data's story.
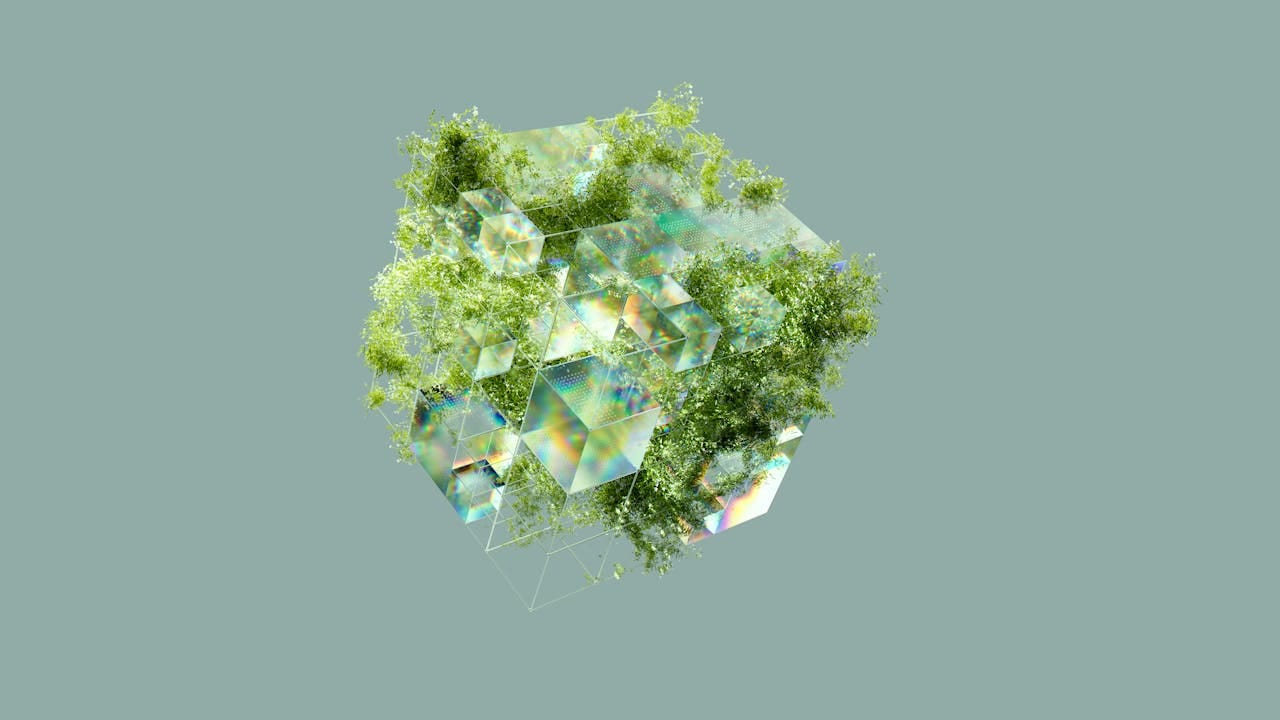
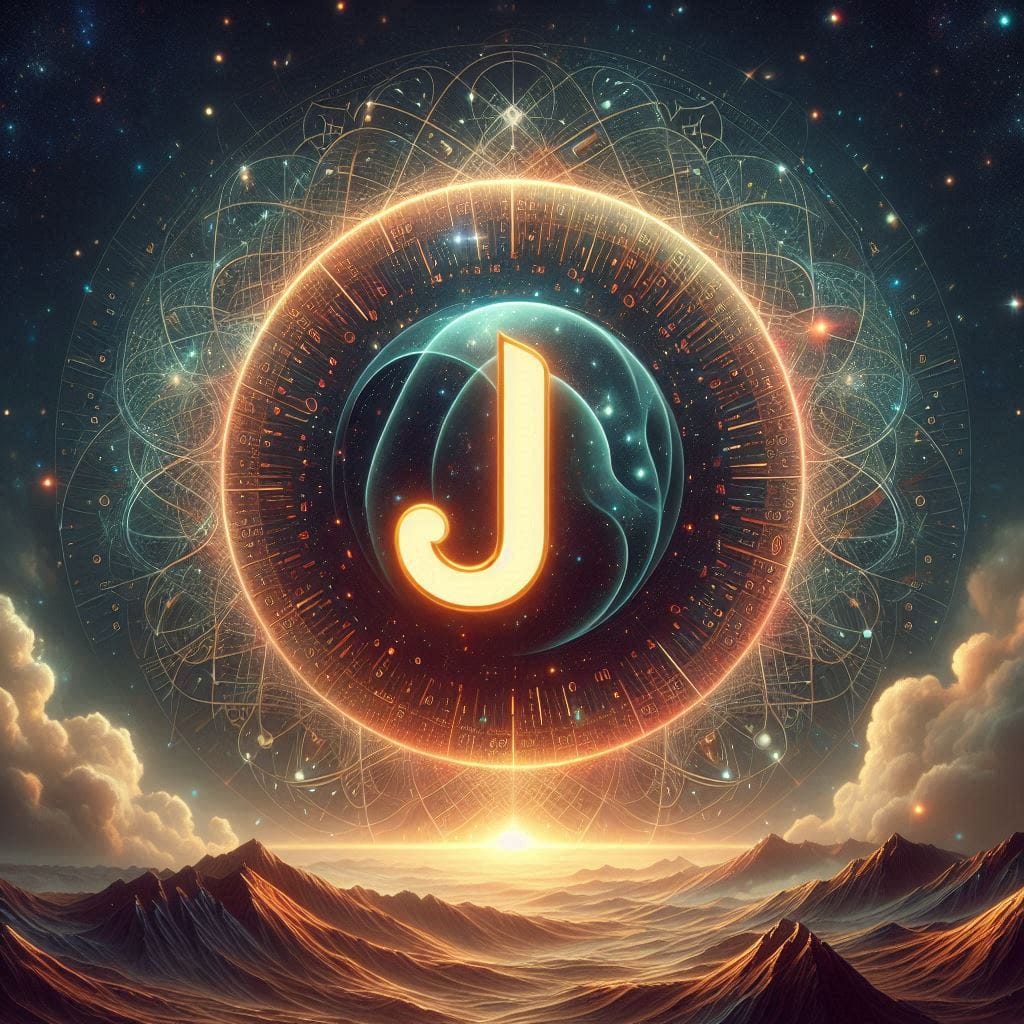